Creating a Simple Budget Tracker App Using React and JavaScript
Written on
Chapter 1: Introduction to Budget Tracker Apps
In this guide, we will explore how to build a budget tracker application utilizing React and JavaScript. This framework is known for its user-friendliness in developing front-end applications.
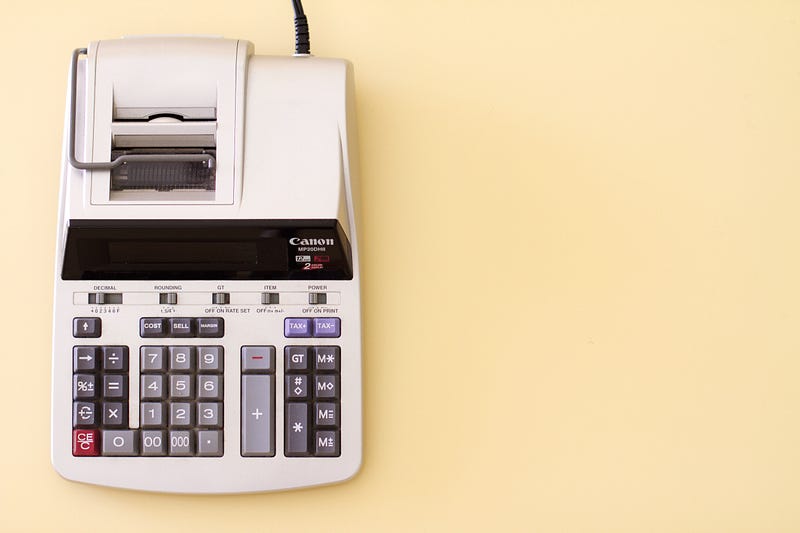
Setting Up the Project
To initiate our React project, we will employ Create React App. You can set this up by running the following command in your terminal:
npx create-react-app budget-tracker
Additionally, we will need the uuid library for generating unique IDs for our budget entries. Install it using:
npm install uuid
Building the Budget Tracker App
We start by importing the necessary hooks from React and the uuid package. Here’s a basic structure of our app:
import { useMemo, useState } from "react";
import { v4 as uuidv4 } from "uuid";
export default function App() {
const [budget, setBudget] = useState("");
const [expense, setExpense] = useState({});
const [expenses, setExpenses] = useState([]);
const add = (e) => {
e.preventDefault();
const { cost, description } = expense;
const formValid = +budget > 0 && +cost > 0 && description;
if (!formValid) {
return;}
setExpenses((ex) => [
...ex,
{
id: uuidv4(),
...expense
}
]);
};
const remove = (index) => {
setExpenses((expenses) => expenses.filter((_, i) => i !== index));};
const remainingBudget = useMemo(() => {
const totalExpenses = expenses
.map(({ cost }) => +cost)
.reduce((a, b) => +a + +b, 0);
return budget - totalExpenses;
}, [budget, expenses]);
return (
<div className="App">
<div>
<form>
<fieldset>
<label>Budget</label>
<input value={budget} onChange={(e) => setBudget(e.target.value)} />
</fieldset>
</form>
<form onSubmit={add}>
<h1>Add Expense</h1>
<fieldset>
<label>Description</label>
<input
value={expense.description}
onChange={(e) =>
setExpense((ex) => ({ ...ex, description: e.target.value }))}
/>
</fieldset>
<fieldset>
<label>Cost</label>
<input
value={expense.cost}
onChange={(e) =>
setExpense((ex) => ({ ...ex, cost: e.target.value }))}
/>
</fieldset>
<button type="submit">Add Expense</button>
</form>
<p>Remaining Budget: ${remainingBudget}</p>
{expenses.map((ex, index) => {
return (
<div key={ex.id}>
{ex.description} - ${ex.cost}
<button onClick={() => remove(index)}>Remove</button>
</div>
);
})}
</div>
</div>
);
}
In this code, we establish three state variables: budget, expense, and expenses. The budget holds the total budget amount, expense stores individual expense entries, and expenses maintains a list of all expenses.
The add function allows for the submission of new expense items while validating the input. If everything checks out, it appends the new expense to the existing array. The remove function enables the deletion of expenses from the list by filtering out the selected item.
The remainingBudget is calculated using the useMemo hook to efficiently compute the remaining balance after accounting for all expenses.
Conclusion
In summary, we have successfully built a simple budget tracker application using React and JavaScript. This app can help users manage their finances effectively.
Chapter 2: Video Tutorials
To further enhance your understanding of building a budget app, check out these helpful video tutorials:
This video tutorial walks you through the steps of creating a budget application with React, covering key concepts and practical implementations.
In this video, you'll learn how to set up a budgeting app using React Router, focusing on the introductory setup and features.