Harnessing the Power of Rust Macros: A Comprehensive Guide
Written on
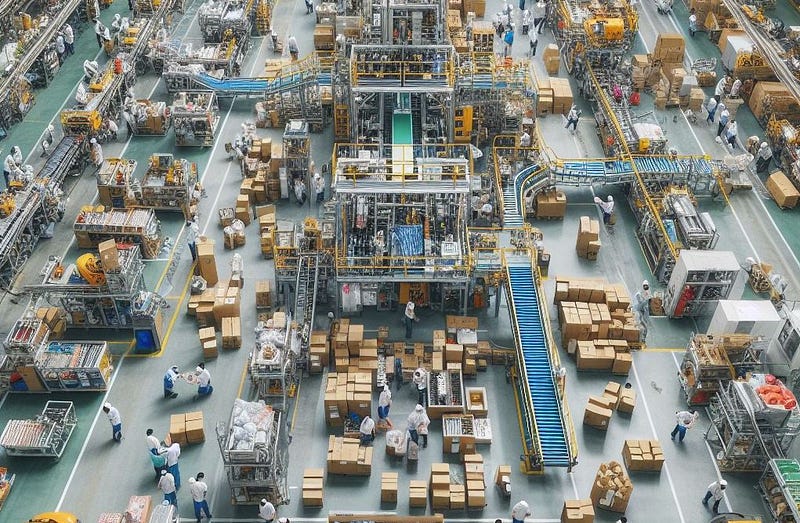
Macros in Rust serve as a robust feature enabling code execution at compile time. They facilitate code generation, provide syntactical abstraction, and allow for compile-time operations, resulting in optimized code.
To define a macro, use the macro_rules! syntax. The definition includes a set of rules, where each rule outlines a specific application of the macro and the corresponding code it should generate. Upon invocation, the compiler looks for the first matching rule and expands the macro according to the defined code.
Here’s a simple example of a macro definition:
macro_rules! add {
($a:expr, $b:expr) => {
$a + $b};
}
This example introduces a macro named add, which contains a single rule. The rule accepts two parameters, $a and $b, binds them to expressions, and outputs their sum.
To use this macro, you would write:
let result = add!(1, 2);
This expands to:
let result = 1 + 2;
Resulting in result being assigned the value of 3.
Rust macros are classified into three primary types:
- Function-like macros: These behave similarly to functions. The add! macro exemplifies a function-like macro.
- Attribute macros: These are used as attributes, such as #[derive(Debug)].
- Derive macros: A subtype of attribute macros that generate trait implementations, like #[derive(Debug)].
Next, we will delve into specific examples of each macro category.
Fundamental Rules of Macros
Rust macros adhere to strict regulations to ensure they remain hygienic (they do not inadvertently capture variables incorrectly) and expand predictably.
#### Macro Hygiene
Hygienic macros prevent unintended variable capture from their surrounding context. This feature is crucial, as it ensures that the behavior of a macro remains consistent, irrespective of the local variables available at the time of use.
By default, Rust macros are hygienic. This means that any variables referenced within a macro are confined to the macro's scope. For instance:
let x = 1;
let y = 2;
macro_rules! multiply {
($a:expr) => {
$a * y // Refers to the localized y, not the outer y};
}
multiply!(x); // Expands to x * y, where y is localized
In this case, the y inside the macro corresponds to the localized y, ensuring that the macro always multiplies by 2, regardless of the outer y.
If you wish to have a macro that captures an outer variable, you need to designate it explicitly using the #[macro_export] attribute:
#[macro_export]
macro_rules! access_outer {
() => {
y // Refers to the outer y};
}
let y = 1;
access_outer!(); // Expands to y (outer y)
Now, the y within the macro refers to the outer variable.
Non-hygienic macros may produce unexpected behaviors and bugs, thus hygienic macros are favored in Rust.
#### Token Stream Manipulation
Macros work by processing Rust code as a stream of tokens. They can:
- Match token patterns (via macro_rules!)
- Consume and replace tokens (using $var)
- Expand recursively
- Return new token streams
This flexibility allows macros to generate complex code with minimal input.
#### Recursive Macros
Rust allows for recursive macros, enabling them to call themselves within their expansion, which is useful for creating repetitive or intricate code.
For example, consider a recursive macro that calculates the factorial of an integer:
macro_rules! factorial {
=> {
1
};
=> {
n * factorial!(n - 1)
};
}
Invoking factorial!(3) expands to:
3 * factorial!(2) // Which further expands to: 3 * 2 * factorial!(1) // And ultimately to: 3 * 2 * 1
It is essential for recursive macros to include a base case to prevent infinite expansion!
Declarative Macros
Declarative macros provide a way to create syntax extensions in a straightforward manner using the macro_rules! construct.
The syntax for defining a declarative macro is as follows:
macro_rules! name {
// macro logic goes here}
For example, we can define a sql_query! macro that generates SQL queries with minimal input:
macro_rules! sql_query {
($table:ident $($col:ident)=$val:expr) => {
format!("SELECT * FROM {} WHERE {}='{}'",
$table,
stringify!($col),
$val)
};
}
This macro can be invoked as follows:
let query = sql_query!(users name="John");
This expands to:
let query = format!("SELECT * FROM users WHERE name='John'");
In this case, $table and $col act as capture identifiers, while $val captures the value expression. The stringify! macro converts the column name into a string at compile time, allowing dynamic insertion of column names into the SQL string.
Declarative macros can be further enhanced to handle:
- Multiple columns
- Various comparison operators
- JOINs
- And more!
The primary advantage of declarative macros lies in their simplicity. They enable the definition of syntax extensions in an accessible way without needing to implement procedural logic.
However, they are limited in scope and may require procedural macros for more complex scenarios.
Procedural Macros
Procedural macros allow the execution of arbitrary Rust code at compile time and are categorized into two types:
#### Attribute Procedural Macros
These macros are applied to items such as functions and structs. They are defined using the proc_macro_attribute crate with the following syntax:
#[proc_macro_attribute]
pub fn my_attribute(args: TokenStream, input: TokenStream) -> TokenStream {
}
The args parameter captures arguments passed to the attribute, while input refers to the item being annotated. The macro must return a TokenStream that represents the modified item.
Here’s an example of a basic custom derive macro:
#[proc_macro_derive(HelloWorld)]
pub fn hello_world(input: TokenStream) -> TokenStream {
let ast = syn::parse(input).unwrap();
impl_hello_world(&ast)
}
fn impl_hello_world(ast: &syn::DeriveInput) -> TokenStream {
let name = &ast.ident;
let gen = quote! {
impl HelloWorld for #name {
fn hello_world() {
println!("Hello, World! My name is {}", stringify!(#name));}
}
};
gen.into()
}
This macro can be utilized like a built-in derive:
#[derive(HelloWorld)]
struct MyStruct;
fn main() {
MyStruct::hello_world();}
#### Function Procedural Macros
Function procedural macros resemble function calls and utilize the proc_macro crate with the syntax:
#[proc_macro]
pub fn my_macro(input: TokenStream) -> TokenStream {
}
For instance, here’s a macro that increments all numbers in an expression by 1:
#[proc_macro]
pub fn increment_numbers(input: TokenStream) -> TokenStream {
increment_numbers_impl(input.into_iter())}
fn increment_numbers_impl(mut iter: token::IntoIter) -> TokenStream {
let mut output = String::new();
while let Some(tt) = iter.next() {
match tt {
token::TokenTree::Literal(lit) => {
if let Ok(num) = lit.to_string().parse::<i32>() {
output.push_str(&(num + 1).to_string());} else {
output.push_str(&lit.to_string());}
}
_ => output.push_str(&tt.to_string()),
}
}
TokenStream::from_str(&output)
}
You can invoke this macro like a function:
let expr = increment_numbers!(1 + 2 * 3);
assert_eq!(expr, "2 + 3 * 4");
Best Practices for Macros
When crafting Rust macros, adhering to certain best practices is crucial for ensuring robustness and compatibility within the Rust ecosystem.
- Avoid Side Effects: Keep your macros pure by only manipulating input and output tokens. Side effects may lead to unpredictable interactions with surrounding code.
- Prefer Declarative Over Procedural: Declarative macros are easier to understand and work more seamlessly with the compiler. Use procedural macros only when necessary.
- Thorough Testing: Write integration tests to check your macros in various contexts and inputs to ensure correctness.
- Choose Descriptive Names: Since macros introduce new syntax, naming them effectively is important. Follow standard naming conventions.
- Organize with Modules: Group related macros into logical modules for easier navigation.
- Avoid Capturing Variables: Macros expand at compile time, so avoid capturing outer variables to prevent unexpected behaviors.
- Utilize Built-in Macros for Strings: Prefer concat! and stringify! for string manipulation instead of traditional concatenation to manage edge cases effectively.
By following these guidelines, you can create robust and useful Rust macros that contribute positively to the programming ecosystem. If you have any further questions, feel free to ask!
I hope you found this guide informative! If it helped you, please consider showing your support!