Mastering Backtesting of Trading Strategies in Python
Written on
Chapter 1: Introduction to Backtesting
Backtesting trading strategies is an essential part of developing and refining trading algorithms. Python has become a favored choice for this purpose because of its user-friendly syntax and versatility. This guide will take you through the process of creating a basic backtesting script with the provided code.
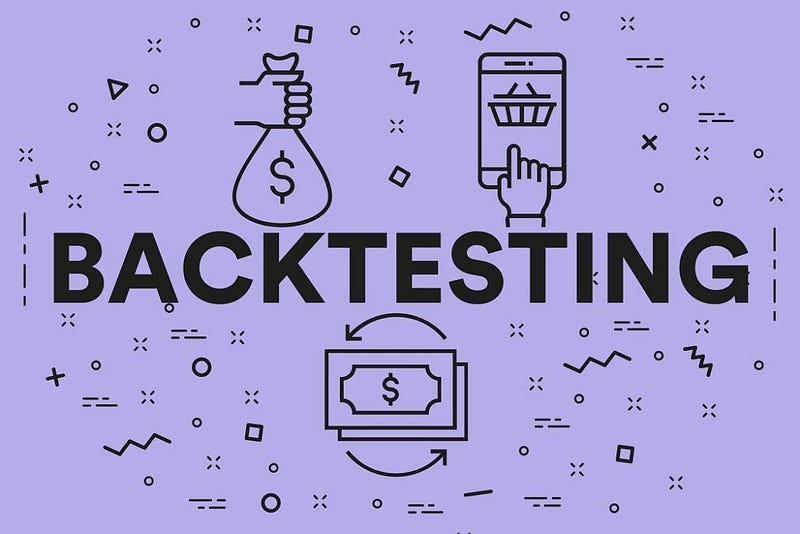
Section 1.1: Setting Up Your Environment
To kick things off, we need to import the libraries that will help us with our code. For our example, we will utilize pandas for efficient data handling.
import pandas as pd
Section 1.2: Data Loading
The next step is to load our historical price data into the script. We can utilize pandas to read data from a CSV file.
df = pd.read_csv('data.csv')
Subsection 1.2.1: Preparing the Data
We will clean our data by dropping any NaN values and replacing infinite values with NaN.
df = df.dropna()
df.replace([np.inf, -np.inf], np.nan, inplace=True)
signals = [0]
As we iterate through the dataset, we will define our trading strategy. For instance, if the STORSI value is above 0.2, we will append a sell signal; if it is below 0.8, we will append a buy signal.
for i in range(1, len(df) - 1):
if df.iloc[i]['STORSI'] > 0.2:
signals.append(-1)elif df.iloc[i]['STORSI'] < 0.8:
signals.append(1)else:
signals.append(0)
signals.append(0)
df["signal"] = signals
Chapter 2: Simulating Trades
Next, we initialize variables to track our investments, including the initial investment amount, current investment, fees, profits, and trading positions.
investment = 1000
current_investment = investment
invested_amount = 0
fees = 0
profit = 0
is_invested = 0
best_trade = float('-inf')
worst_trade = float('inf')
largest_loss = 0
largest_gain = 0
total_trades = 0
We can now iterate through our historical data to simulate the trades according to our strategy.
for i in range(500, len(df)):
signal = df.iloc[i]['signal']
close = df.iloc[i]['close']
if signal == 1 and is_invested == 0:
entry_point = close
quantity = (current_investment / close)
invested_amount = quantity * close
is_invested = 1
elif signal == -1 and is_invested == 0:
entry_point = close
quantity = (current_investment / close)
invested_amount = quantity * close
is_invested = -1
elif signal == -1 and is_invested == 1:
profit = quantity * (-entry_point + close)
current_investment += profit
invested_amount = 0
total_trades += 1
largest_gain = max(largest_gain, profit)
largest_loss = min(largest_loss, profit)
best_trade = max(best_trade, profit)
worst_trade = min(worst_trade, profit)
entry_point = close
quantity = (current_investment / close)
invested_amount = quantity * close
is_invested = -1
elif signal == 1 and is_invested == -1:
profit = quantity * (-close + entry_point)
current_investment += profit
invested_amount = 0
total_trades += 1
largest_gain = max(largest_gain, profit)
largest_loss = min(largest_loss, profit)
best_trade = max(best_trade, profit)
worst_trade = min(worst_trade, profit)
entry_point = close
quantity = (current_investment / close)
invested_amount = quantity * close
is_invested = 1
else:
pass
Final results can then be printed out to evaluate performance.
final_profit = current_investment - investment
print("Final Profit: ", final_profit)
print("Best Trade: ", best_trade)
print("Worst Trade: ", worst_trade)
print("Largest Loss: ", largest_loss)
print("Largest Gain: ", largest_gain)
print("Total Trades: ", total_trades)
In summary, backtesting is vital for the development and assessment of trading strategies. Python offers a robust set of libraries and tools that simplify the backtesting process. The code illustrated in this guide serves as a foundational strategy, which can be expanded upon for more intricate methods.
It is crucial to approach backtesting results with caution, ensuring they are validated through out-of-sample testing and other evaluation metrics. With the right analysis, backtesting can significantly enhance traders' and investors' abilities to refine their strategies and make well-informed decisions.
Chapter 3: Additional Resources
To further enhance your understanding of backtesting in Python, consider watching the following videos:
This video offers a step-by-step tutorial on backtesting trading strategies using Python and Visual Studio Code, making it a valuable resource for beginners.
This video explains how to backtest a trading strategy in Python, providing insights into best practices and methodologies for effective trading.